- COMP.CS.140
- 12. Graphical User Interfaces
- 12.4 ⌛⌛⌛ JavaFX Wordle
⌛⌛⌛ JavaFX Wordle¶
Place the main class of your implementation into the file
Round12/wordle/src/main/java/fi/tuni/prog3/wordle/Wordle.java
. As implied by the directory
structure, the implementation must belong to the package fi.tuni.prog3.wordle
.
In this task you should create a JavaFX-based implementation of the Wordle word game that has gained some media attention recently.
The game is in many ways similar to the word game implemented in an earlier exercise task. The game proceeds as follows:
The game master chooses a word (that is not shown to the player).
The original Wordle uses words of fixed length 5. In this task the word length may vary.
The goal of the player is to guess the game master’s word by doing at most 6 guesses.
Each guess involves guessing the whole word. The game master will after each guess describe which letters in the guess occur in the game master’s word (more about this further below).
The GUI and other implementation details must follow the guidelines given below. The guideline includes some example GUI images. Note that these images are meant as examples, that is, the GUI implemented by you can have a different appearance as long as it offers similar functionality. But the rules about cell colors must be followed precisely because the automated grader will check cell colors.
When the game starts, the GUI shows an empty grid that has 6 rows and whose number of columns corresponds to the length of the game master’s word.
Each row corresponds to a single guess: the first row to the first guess, the second row to the second guess, and so on.
The grid cells are initially empty (contain the empty string “”) and their background must be white (
Color.WHITE
).
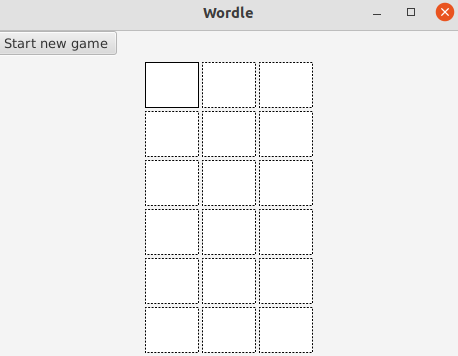
An example of the game’s initial state.¶
The player composes a guess by typing letters on the keyboard.
Letters are handled as uppercase. If the player types a lowercase letter, the game will automatically convert it to uppercase.
You should handle also the game master’s word as all-uppercase when checking a player’s guess (so the game will work in a case-insensitive manner).
Each typed letter will be inserted into the first empty grid cell on the row corresponding to the current guess.
If the row is already full, attempts to type more characters will not affect the cells.
The player can remove previously typed letters by using the backspace key. Each press of the backspace key will remove the last letter (if such exists) on the row corresponding to the current guess.
Removing a letter means that the corresponding cell will be set to contain the empty string “”. Do not use e.g.
null
.
The player submits a guess for checking by pressing the Enter key.
If the player presses Enter before the current guess row is full, the game should show the message “
Give a complete word before pressing Enter!
” to the player.
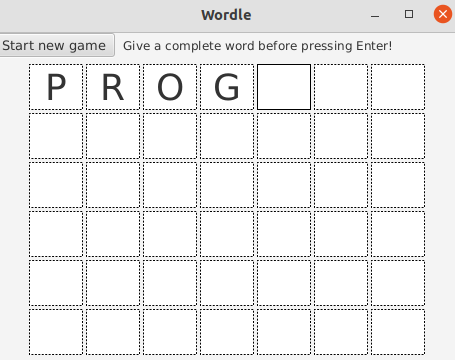
An example where the player has pressed Enter when the guess is still incomplete.¶
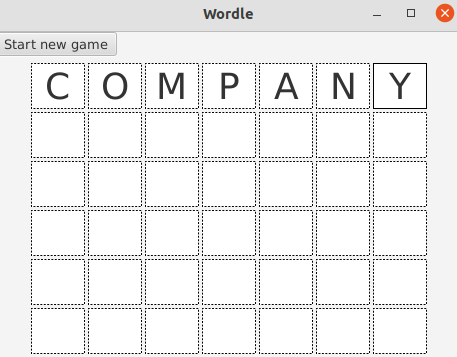
An example where the player has typed a complete guess without having yet pressed Enter.¶
The game indicates the correct parts of the player’s guess by coloring the cells on the current guess row as follows:
If a letter in the guess matches with the letter in the corresponding position of the game master’s word, the letter’s cell will be colored green (
Color.GREEN
).Otherwise, if a letter in the guess matches with a letter in some other position of the game master’s word, the letter’s cell will be colored orange (
Color.ORANGE
).Otherwise, a letter that does not match any letter in the game master’s word will have its cell colored grey (
Color.GREY
).
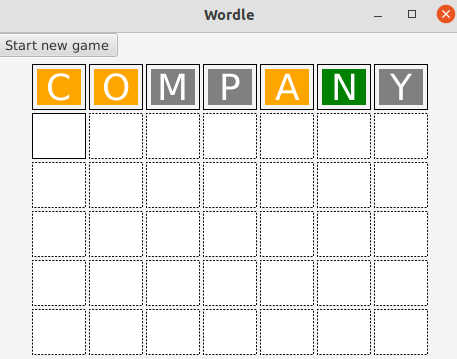
An example where the player has typed a complete guess and then pressed Enter. Here the game
master’s word is “account
”. The cell of the exactly matching letter ‘N
’ was colored
green, the cells of the misaligned matching letters ‘C
’, ‘O
’ and ‘A
’ were colored
orange, and the cells of the completely non-matching letters ‘M
’, ‘P
’ ja ‘Y
’ were
colored grey.¶
If the player guessed the word correctly, the message “
Congratulations, you won!
” is shown to the player and the current game ends. If the player’s guess was incorrect and it was the 6th guess, the message “Game over, you lost!
” is shown to the player and the current game ends.The player cannot type new letters (and Enter will do nothing) when the current game has ended.
The player can start a new game by clicking the button “
Start new game
”.
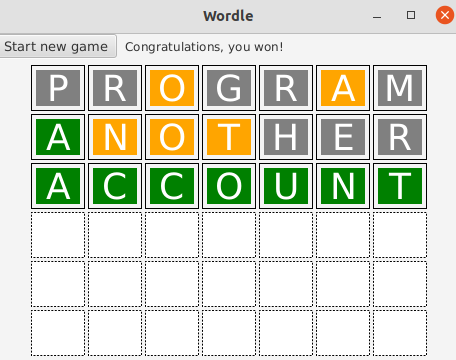
An example where the player guessed the game master’s word and won the game.¶
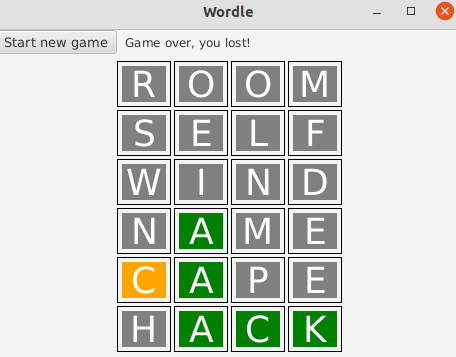
An example where the player made 6 wrong guesses and lost the game.¶
If the player’s guess did not end the game, the game will continue from the beginning of the next grid row: new letters typed by the player will start to compose a new guess on the next row.
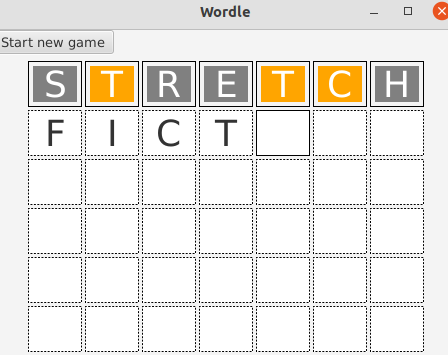
An example where the player is composing the next guess.¶
The above description corresponds more or less to how the original Wordle game works.
The main class of your implementation must be fi.tuni.prog3.wordle.Wordle
(that is, the class
Wordle
belonging to the package fi.tuni.prog3.wordle
). Your implementation can contain also
other source files as long as also they are located in the directory
src/main/java/fi/tuni/prog3/wordle
.
Some further requirements related to GUI elements and selecting the game master’s words:
The program must initially read a list of game master’s words from the file
words.txt
and initialize a game that uses the first read word. The file is expected to contain one word per line.You may test your program with the word file provided in the previous word game exercise task. In that case you program should first initialize a game that uses “
account
” as the game master’s word.
If the player clicks the button “
Start new game
”, the program should select the next word from the list of game master’s words and initialize a new game using it. In case of the word file mentioned above, the next word would be “act
”.The grid is again initialized to contain empty grid cells. Note that the grid size may change as the length of the new game master’s word can be different.
In order to facilitate automated unit testing, the GUI elements need to have id values described below. JavaFX GUI elements have a member function
setId
for setting an id.The cell in column
j
of rowi
must have an id of form “i_j”. We assume 0-based indexing.Note: the id must begiven to the element that directly contains the text representing the cell’s letter.
E.g. the cells on the first (top) grid row would have the id’s “0_0”, “0_1”, and so on. If the game master’s word has e.g. length 7, the bottom-right corner cell (in column 6 of row 5) would have the id “5_6”.
The element directly containing messages shown to the player (e.g. about the game result or pressing Enter prematurely) must have the id “infoBox”.
The “
Start new game
” buitton must have the id “newGameBtn”.
The elements that directly contain the cell letters, and the element that directly contains messages to the player, must be chosen from the JavaFX element types
Text
,TextField
,TextArea
,Label
,Cell
andButton
.
About testing¶
Due to using a GUI, this task does not have example input or output files. You should test your
program yourself by e.g. comparing the behaviour of your program with the example images given
above. All example images where the game master’s word has length 7 correspond to the word
“account
”.
The task submission will be opened later after some further preparation. The automated tests will perform unit tests that reward points in an accumulative manner: the more tests your program passes, the more points you will get. The task will thus award also partial points.
A+ presents the exercise submission form here.