- COMP.CS.200
- 5. JavaScript: basics + tutorial (assignment)
- 5.3 Update HTML text content with JavaScript
Update HTML text content with JavaScript¶
Attention
This exercise should be done in groups and submitted as a group.
Include all group members and make sure that everyone knows how your solution works. Only one group member needs to submit the exercise on behalf of the whole group.
These exercises require JavaScript and you should know some basics of it before you can complete these exercises. So make sure you know the basics of JavaScript. The first reference below should be easy to understand and follow. The first two chapters are probably enough for you to be able to complete these exercises but if not then you now know where to find more JavaScript info. The Mozilla Developer Network (MDN) tutorial is a bit more technical and assumes some prior knowledge of programming but it is still a very good quick reference now or later on when you feel more confident of your skills.
Background Information¶
Completing these exercises requires some background knowledge of how JavaScript works in the browser and how the webpage user can interact with JavaScript. There are two main concepts that you need to know here. The Document Object Model (DOM) and events.
Document Object Model (DOM) is JavaScript’s representation of the HTML and all elements in the page. Every element and its attributes as well as all the styles and text contents of each element are stored in a hierarchical tree structure. JavaScript can therefore access and manipulate all of the document contents and make changes to the styles and texts of the elements and it can also create and remove elements.
Events on the other hand are the basis of any interactivity on any web page. The browser generates events every time something happens that can or will have an effect on the document. These events include mouse clicks, keyboard events such as pressing down a key and releasing a key, scrolling page up or down, submitting a form, changing browser’s window size (viewport) and so on. JavaScript code can then attach an event listener or several listeners to any and all of these events as needed. Event listeners are functions that receive an Event object describing the details of the event that occurred and the event listener can then do creative things that you all have seen many pages perform around the internet. These things include validating a form input and showing an error message if form is not filled correctly or showing and hiding elements based on user actions but they can also be very complicated things such as drawing graphics on the screen and moving that graphics around based on user’s actions as in games and animations.
Instructions¶
In this exercise, we update the content of an HTML page with JavaScript. You are going to build a form handler that takes the user’s input and replaces the previous content of a paragraph with the given input.
Download the template¶
Instructions¶
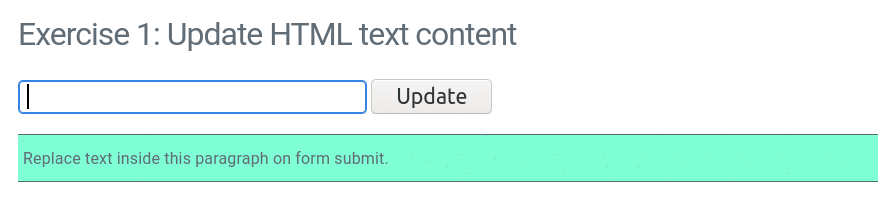
In the image above, you see the anticipated end result. After you have typed some text to the input field and submitted the form, the input replaces the content of the paragraph and the input field is reset back to empty to be ready for next input.
NOTE: The stages below are not all required and the end result is what counts. For more experienced JavaScript coders some of the stages may seem unnecessary but for others they should prove helpful when there are a few checkpoints included in between and some functions are built incrementally.
1. First start by downloading the template files. The HTML template takes care of loading the
JavaScript file and you don’t have to change anything else but the index.js
file. All your code goes
inside the index.js
file and this is the only file you submit in this exercise.
2. In the HTML file there are three important elements: the form input which has id “type-input”, the target
paragraph which has an id “receive-input” and the form itself which has an id “form”. Your first task is to
get a hold of all these elements inside your code. In JavaScript you can get to a specific element in many
different ways but here the fact that all those element have an ID helps us since IDs are always unique and you
can find a specific element based on its ID. You can use document.getElementById()
or document.querySelector()
which both return only one element provided that there exists an element that fulfills the search criteria.
For example the form element could be found either with document.getElementById("form")
or document.querySelector("#form")
.
The first of these two methods does exactly what you would guess, it gets an element by its ID but the second one
is more interesting since it accepts a CSS selector as it argument and you can find also elements that do not have
an ID and here we have to add that “#” hash character in front of the id just like in CSS. Interestingly in this case it
would also work simply with document.querySelector("form")
but the difference is the same as in CSS that now
we refer to all form elements and not just the one but since document.querySelector() always returns the first matching
element in this case that would work just fine. There is also a method document.querySelectorAll()
that would
return all the matched elements and not just the first one.
See: Searching: getElement*, querySelector*
3. Next implement the submitHandler(e)
function that is inside the index.js file. This function reads the
value of the input field and replaces the contents of the paragraph with the value but only if the value is not empty
and contains more than just spaces. Then it resets the input fields value back to empty string. Replacing element’s
contents with text can easily be done by setting the element’s textContent
property to be the desired text.
This function takes one argument named e
, which is the submit event from the browser.
4. Next attach the function you just created to the submit event of the form. You can now try and test your form.
You will immediately find out that your function does not appear to work and the text inside the paragraph is not
replaced but the input field is still reset to empty. This is because the browser has a default action of its own
when a form is submitted and in this case the browser is actually sending the form and its data back to the URL
address defined by the form’s action-attribute or if it is not present the browser assumes it to be the same
address the original document was loaded from. In any case this means that the browser loads a new page
(or in our case the same page). This loads the page again instead of replacing the text. Your event handler should
cancel that default action before modifying the document. This is where the argument e comes in. That is the submit
event and you should add e.preventDefault()
as the first line in your function.
See: Forms: event and method submit (By the way, although
not all events have default actions associated with them one another example of a default action is
when you click a link and the browser follows the link and loads a new page.
See: Browser default actions)
5. Reload your page to make sure that your changes to the JavaScript file are loaded and test your application. Try inputting some text and submitting the form and see if the paragraph text is replaced correctly and the input field is reset to empty. Try this a few times. If everything works congratulations! Otherwise you should see some error messages in the browser console. Depending on the message you should try to locate the source of the error and fix it. In any case the error message should include the line number of the error. See: Debugging in Chrome
A+ presents the exercise submission form here.