Testing Framework JUnit¶
NetBeans creates the JUnit testcode for a Java file from Tools->Create/Update Tests.
The basic structure of a JUnit test file is:
Unit tests are written using a test framework.
For Java on such framework is JUnit which is intented for test driven development.
The latest version, JUnit 5, is in the package org.junit.jupiter
.
The earlier versions JUnit 4 and JUnit 3 are in packages org.junit
and junit.framework
respectively.
The user guide for JUnit is available online: JUnit User Guide.
NetBeans offers support for JUnit Jupiter and the JUnit Platform as a part of the IDE. Visual Studio Code supports JUnit Jupiter and the JUnit Platform via the Java Test Runner extension which is installed by default as part of the Java Extension Pack.
A JUnit test fixture is a Java object.
In the code of the test class, the test methods are annotated with the @Test
annotation.
If needed, it is also possible to define a method to execute before (or after) each (or all) of the test methods with the @BeforeEach
(or @AfterEach
) and @BeforeAll
(or @AfterAll
) annotations.
A list of JUnit annotations.
NetBeans creates the JUnit test code for Java class files from the menu Tools->Create/Update Tests. The basic structure of the tes class is:
import org.junit.jupiter.api.AfterEach;
import org.junit.jupiter.api.AfterAll;
import org.junit.jupiter.api.BeforeEach;
import org.junit.jupiter.api.BeforeAll;
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.*;
public class MyClassTest {
@BeforeAll
public static void setUpClass() throws Exception {
// Actions executed before the first test case
}
@BeforeEach
public void setUp() throws Exception {
// Actions executed before each test case
}
@Test
public void testMethod() {
// Code for testing a method
}
@AfterEach
public void tearDown() throws Exception {
// Actions executed after each test case
}
@AfterAll
public static void tearDownClass() throws Exception {
// Actions executed after the last test case
}
}
In addition different conditions can be defined for running the tests, tests can be parameterized, a specific execution order can be defined, a specific amount of executions for a test can be defined etc.
An example of a test class testing the Date
class:
public class DateTest {
@Test
public void testGetYear() {
// print the name of the method
System.out.println("getYear");
// create an instance of the tested class
Date instance = new Date(2022, 2, 28);
// define the expected output
int expResult = 2022;
// fetch the state of the tested instance
int result = instance.getYear();
// check is the fetched state matches the expected output
// if not assertEquals throws AssertionError
assertEquals(expResult, result);
}
@Test
public void testGetMonth() {
System.out.println("getMonth");
Date instance = new Date(2022, 2, 28);;
int expResult = 2;
int result = instance.getMonth();
assertEquals(expResult, result);
}
@Test
public void testGetDay() {
System.out.println("getDay");
Date instance = new Date(2022, 2, 28);;
int expResult = 28;
int result = instance.getDay();
assertEquals(expResult, result);
}
@Test
public void testToString() {
System.out.println("toString");
Date instance = new Date(2022, 2, 28);;
String expResult = "28.02.2022";
String result = instance.toString();
assertEquals(expResult, result);
}
@Test
public void whenExceptionThrown() {
System.out.println("whenExceptionThrown");
Exception exception = assertThrows(DateException.class, () -> {
new Date(2022, 2, 29);
});
String expectedMessage = "Illegal date 29.02.2022";
String actualMessage = exception.getMessage();
System.out.println(actualMessage);
assertTrue(actualMessage.contains(expectedMessage));
}
// making a parameterized test
// input is given with the method intProvider
@ParameterizedTest
@MethodSource("intProvider")
void testLegalDates(int year, int month, int day) {
System.out.println("isLegalDate");
assertTrue(Date.isLegalDate(day, month, year));
}
// A method returning the parameters for the test as a stream
// In addition to a stream any collection such as List could be used
static Stream<Arguments> intProvider() {
return Stream.of(
arguments(2014, 2, 14),
arguments(2020, 5, 30)
);
}
}
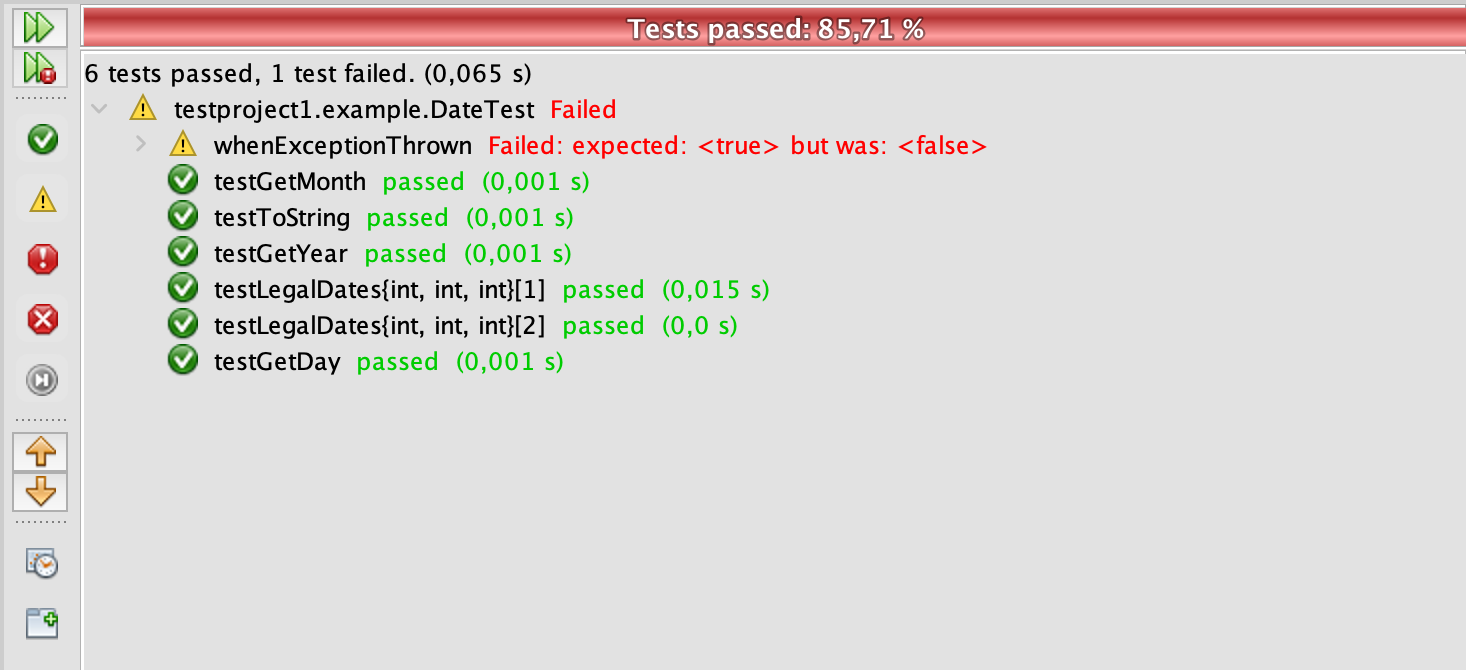
The result of the tests and the reason if failed is shown for each test¶