⌛ JavaFX calculator¶
Place the main class of your implementation into the file
Round12/calc/src/main/java/fi/tuni/prog3/calc/Calculator.java
. As implied by the directory
structure, the implementation must belong to the package fi.tuni.prog3.calc
.
This task concerns implementing a simple calculator with the JavaFX GUI library. The calculator must have the following parts:
Input fields for the first and second operand.
The input fields must be JavaFX
TextField
elements that have id’s “fieldOp1
” and “fieldOp2
”. Set the id’s by using the JavaFX element member functionsetId
.The input fields must be preceded by the label texts “
First operand:
” and “Second operand:
”. These must be implemented either as JavaFXText
orLabel
elements and have id’s “labelOp1
” and “labelOp2
”.
Buttons for the four basic arithmetic operations: addition, subtraction, multiplication and division.
These must be JavaFX
Button
elements and have id’s “btnAdd
”, “btnSub
”, “btnMul
” and “btnDiv
”.The buttons must have texts “
Add
”, “Subtract
”, “Multiply
” and “Divide
”.
A result field.
The result field must be a JavaFX
Label
element that has the id “fieldRes
” and whose background is white (see e.g. the documentation of the member functionsetBackground
; you may use the colorColor.WHITE
). The result field is initially empty (contains “”).The result field must be preceded by the label text “
Result:
” implemented either as JavaFXText
orLabel
element whose id is “labelRes
”.
You have to specify the above-mentioned element id’s in order to allow the grader to use simple unit tests in testing your GUI implementation.
Below is an example image of a calculator GUI. Note that this is an example: you do not have to implement an identical GUI. Just make sure that your GUI contains the required parts in some form and layout.
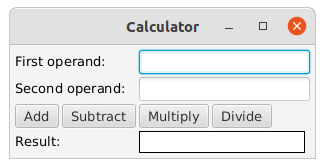
If a user sets values into the operator input fields and presses one of the buttons, the result of
applying the the corresponding operation to the two operands should appear in the result field.
The operation will not affect the contents of the input fields; they will hold their existing
values until the user explicitly modifies them. The program must use double
variables for
handling the input values and the result. Below are examples of all four operations:
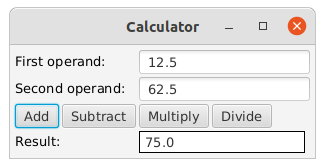
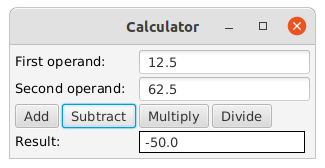
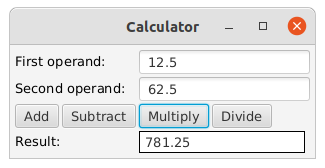
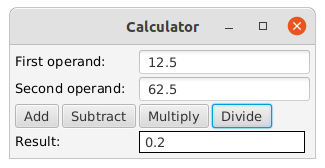
The main class of your implementation must be fi.tuni.prog3.calc.Calculator
(that is, the class
Calculator
belonging to the package fi.tuni.prog3.calc
). By a “main class” we mean the
class that contains your implementation of the function start
that initializes the JavaFX GUI.
Your implementation can contain also other source files as long as also they are located in the
directory src/main/java/fi/tuni/prog3/calc
.
About testing¶
Due to using a GUI, this task does not have example input or output files. But it should be fairly simple to test the calculator on your own; the calculator has a quite limited functionality and the operations are simple to compute. Be sure to check that you have not made typos when setting the element id’s and label texts.
The task submission will be opened later after some further preparation. The automated tests will perform unit tests that reward points in an accumulative manner: the more tests your program passes, the more points you will get. The task will thus award also partial points.
A+ presents the exercise submission form here.