- COMP.CS.200
- 6. JavaScript: DOM, events, objects (assignment)
- 6.5 Co-authors
Co-authors¶
Attention
This exercise should be done in groups and submitted as a group.
Include all group members and make sure that everyone knows how your solution works. Only one group member needs to submit the exercise on behalf of the whole group.
Again you will write functions which take data as a JavaScript object and manipulate it to give it a different representations.
In the previous two exercises you were using data from Stack Overflow yearly user surveys but this time you will use publications data from the dblp computer science bibliography.
Because of the huge amount of data in the database we have used search terms “javascript” and “functional” to limit the data to publications which include both of those words in their title.
NOTE: You need a couple of functions from the previous exercises here too. Finish them first!!
Download the templates¶
Instructions¶
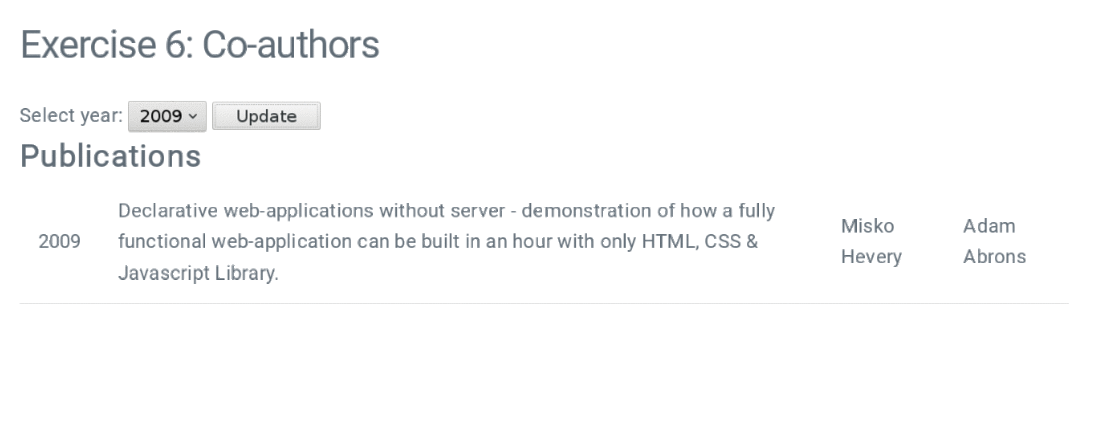
Begin by downloading and extracting the exercise files. You should have the following four files:
coauthors.js
this file is for your functions. You will edit and submit only this file.index.html
this file is responsible for loading all the other files and showing the final results.style.css
stylesheet to make the HTML look a bit nicer than the browser defaults.publ.js
this file contains the publications data. The structure of the data is shown below (although there will be more data):const publicationsData = [ { _id: '716991', authors: { author: [ { '@pid': '281/4693', text: 'Alexander Sobolev' }, { '@pid': '49/5572', text: 'Sergey Zykov' } ] }, title: 'Functional Programming Patterns in JavaScript.', venue: 'KES-IDT', pages: '299-312', year: '2019', type: 'Conference and Workshop Papers', key: 'conf/kesidt/SobolevZ19', doi: '10.1007/978-981-13-8311-3_26', ee: 'https://doi.org/10.1007/978-981-13-8311-3_26', url: 'https://dblp.org/rec/conf/kesidt/SobolevZ19' }, { _id: '1478685', authors: { author: [ { '@pid': '197/7003', text: 'Lukás Janecek' }, { '@pid': '94/6230', text: 'Robert Pergl' } ] }, title: 'Analysing Functional Paradigm Concepts - The JavaScript Case.', venue: 'WorldCIST', pages: '882-891', year: '2017', type: 'Conference and Workshop Papers', key: 'conf/worldcist/JanecekP17', doi: '10.1007/978-3-319-56535-4_86', ee: 'https://doi.org/10.1007/978-3-319-56535-4_86', url: 'https://dblp.org/rec/conf/worldcist/JanecekP17' }, { _id: '1535634', title: 'React - Facebook's functional turn on writing Javascript.', venue: 'Commun. ACM', volume: '59', number: '12', pages: '56-62', year: '2016', type: 'Journal Articles', key: 'journals/cacm/X16p', doi: '10.1145/2980991', ee: 'https://doi.org/10.1145/2980991', url: 'https://dblp.org/rec/journals/cacm/X16p' }, { _id: '2731234', authors: { author: { '@pid': '39/2573', text: 'Brian McKenna' } }, title: 'Roy - A Statically Typed, Functional Language for JavaScript.', venue: 'IEEE Internet Comput.', volume: '16', number: '3', pages: '86-91', year: '2012', type: 'Journal Articles', key: 'journals/internet/McKenna12', doi: '10.1109/MIC.2012.56', ee: 'https://doi.org/10.1109/MIC.2012.56', url: 'https://dblp.org/rec/journals/internet/McKenna12' } ];
As you can see the object
publicationsData
is an array containing multiple publications. In this exercise we are only interested in the year, title, and authors of the publications.
The image above shows the basic idea of this exercise. You populate a dropdown list (a select element) with the years of the publications. Whenever a selection is made and the user submits the form i.e. clicks the “Update” button that triggers a modification to the table of publications, so that it is showing publications of that year. In the list there is also an option all. If selected, then all publications are shown.
Your task is to implement the following functions described in the
coauthors.js
file:
constructTableRowsHtml(rowData)
Copy your function from previous exerciseconstructTableHeadHtml(headings)
Copy your function from previous exercisegetPublications(publicationsData)
Takes as its only argument the
publicationsData
object frompubl.js
Returns a new object of the form:
{ "2010": [ { "title": "Standardized and Extensible Javascript-API for Logging Functionality for the MVP Player.", "authors": [ { "@pid": "19/7308", "text": "Jörn Heid" }, { "@pid": "164/9686", "text": "Frank Hess" }, { "@pid": "07/7251", "text": "Simone Huber" }, { "@pid": "03/7308", "text": "Martin Haag" } ], "year": "2010" }, { "title": "Virtual browser - a web-level sandbox to secure third-party JavaScript without sacrificing functionality.", "authors": [ { "@pid": "28/8733", "text": "Yinzhi Cao" }, { "@pid": "55/4022", "text": "Zhichun Li" }, { "@pid": "91/8736", "text": "Vaibhav Rastogi" }, { "@pid": "88/2827-4", "text": "Yan Chen 0004" } ], "year": "2010" } ], "2011": [ { "title": "ClojureScript - Functional Programming for JavaScript Platforms.", "authors": [ { "@pid": "47/7087", "text": "Mark McGranaghan" } ], "year": "2011" } ], "2012": [ { "title": "Roy - A Statically Typed, Functional Language for JavaScript.", "authors": [ { "@pid": "39/2573", "text": "Brian McKenna" } ], "year": "2012" } ] }Publications are classified by year. And under each year there is an array of publications from that specific year. For each publication only the title, authors, and year properties are returned.
NOTE: Skip publications where there are no authors listed and for those publications which only have one author convert the value of authors property to an array which contains only that single author object. The value of authors property should always be an array of objects!
getYears(publications)
Takes as its only argument the object from
getPublications(publicationsData)
functionReturns a sorted array of unique years from publications (ascending order) You need to remove any duplicate values from the array before returning it.
The keys of the
publications
object are the years of those publications. You can get an array of all the object keys with Object.keys() See also: JavaScript Object.keys(): A GuideIf you need help with sorting the array, see: JavaScript Array sort: Sorting Array Elements
fillOptionList(years)
Takes as its only argument the years array returned by the
getYears(publications)
functionLoops through the
years
array and builds options for the select element in the HTML template.Each option should be of the following format:
<option value="2011">2011</option>The value is year, and text shown to the user is also the year.
The last element of the list should be an option for selecting all the years and should look like the following HTML:
<option value="all">All</option>NOTE: This function should replace the current options of the select element, and not simply append them to the end of the list.
constructPublicationRowData(publications, years)
Arguments:
publications: object of the same format returned by
getPublications(publicationsData)
years: array of years which should be included in the data
Returns an array of publication row data suitable for
constructTableRowsHtml()
Each row should contain these four items: the year of publication, title of the publication, name of the first author, names of the rest of the authors separated by a commas (,) or empty string if the publication only has single author. Example of the produced rowData:[ [ "2010", "Standardized and Extensible Javascript-API for Logging Functionality for the MVP Player.", "Jörn Heid", "Frank Hess, Simone Huber, Martin Haag" ], [ "2011", "ClojureScript - Functional Programming for JavaScript Platforms.", "Mark McGranaghan", "" ] ]
constructPublicationsTableHtml(publications, year = null)
Construct HTML for publications table based on selected year or all publications if year is not given
Arguments:
publications: object of the same format returned by
getPublications(publicationsData)
year: integer or string representing the selected year or null if all years are selected
Uses
constructPublicationRowData()
to construct the table row data andconstructTableHeadHtml()
andconstructTableRowsHtml()
to build the table HTMLThe headings of the table should be “Year”, “Title”, “The 1st author”, “Co-authors” (without the quotation marks)
The table should have a caption: “Publications” (without the quotation marks) and the head and body should be wrapped inside <thead> and <tbody> elements respectively.
Returns table HTML as a string
init()
This function initializes the application (loads all needed data, updates DOM, attaches event handlers, etc.)
index.html
will only callinit()
function and it is the responsibility of this function to connect everything in a way that the application has the desired functionality as described and shown in the image above. This function does not return anything but uses the other functions to perform the following tasks:
get publications from
publicationsData
get years from publications
fill select options
set table to show publications from the first year in the select list (2009)
attach event handler on the form submit to update the table based on the selection
NOTE: use the div with ID “container” as the target for the table (replace the contents of this div with the table HTML)
NOTE 1: All your code goes inside the coauthors.js
file and this is the only file you submit in this exercise.
NOTE 2: Do not rename the functions or else tests won’t be able to detect and use them.
Open index.html
in your browser. If your functions are implemented correctly
you should be able to select a year from the list and update the table based on your
selection.
A+ presents the exercise submission form here.